PHP Command Line Interface (CLI) offers advanced features and techniques for developers to create powerful scripts and tools. In this guide, we’ll explore more advanced aspects of PHP CLI, including error handling, asynchronous programming, and interacting with APIs.
1. Advanced Error Handling
Custom Error Handlers
Define custom error handlers to handle errors and exceptions in a more controlled manner:
<?php set_error_handler(function ($errno, $errstr, $errfile, $errline) { // Custom error handling logic echo "Error: $errstr in $errfile on line $errline\n"; }); set_exception_handler(function ($exception) { // Custom exception handling logic echo "Exception: " . $exception->getMessage() . "\n"; }); // Your script logic goes here ?>
Logging Errors
Redirect errors to a log file for future analysis:
<?php ini_set('log_errors', 1); ini_set('error_log', '/path/to/error.log'); // Your script logic goes here ?>
2. Asynchronous Programming
Asynchronous Scripting with Amp
Amp is a library for asynchronous programming in PHP. Install it using Composer:
composer require amphp/amp
Use Amp to write asynchronous PHP scripts:
<?php require 'vendor/autoload.php'; Amp\Loop::run(function () { $result = yield Amp\asyncCall(function () { // Asynchronous task yield Amp\delay(1000); // Simulate a delay return "Async task completed"; }); echo $result . "\n"; }); ?>
3. Interacting with APIs
HTTP Requests with Guzzle
Guzzle is a powerful HTTP client for PHP. Install it using Composer:
composer require guzzlehttp/guzzle
Use Guzzle for making HTTP requests:
<?php require 'vendor/autoload.php'; use GuzzleHttp\Client; $client = new Client(); $response = $client->get('https://api.example.com/data'); $data = json_decode($response->getBody(), true); print_r($data); ?>
4. Command Line Arguments Processing
Using getopt()
Use getopt()
to process command line options and arguments:
<?php $options = getopt("f:t:"); if (isset($options['f'])) { echo "From: {$options['f']}\n"; } if (isset($options['t'])) { echo "To: {$options['t']}\n"; } ?>
Run the script with:
php script.php -f value1 -t value2
5. Parallel Processing
Parallel Processing with pthreads
pthreads is a PHP extension that provides multi-threading support. Be sure to check the documentation for installation instructions.
<?php class MyThread extends Thread { public function run() { echo "Thread ID: " . $this->getThreadId() . "\n"; } } $threads = []; for ($i = 0; $i < 5; $i++) { $threads[] = new MyThread(); } foreach ($threads as $thread) { $thread->start(); } foreach ($threads as $thread) { $thread->join(); } ?>
6. Creating Interactive Command Line Tools
Using Symfony Console Component
The Symfony Console Component simplifies creating feature-rich command line tools. Install it using Composer:
composer require symfony/console
Create a command:
<?php // myCommand.php require 'vendor/autoload.php'; use Symfony\Component\Console\Command\Command; use Symfony\Component\Console\Input\InputInterface; use Symfony\Component\Console\Output\OutputInterface; class MyCommand extends Command { protected static $defaultName = 'myCommand'; protected function configure() { $this->setDescription('My custom command') ->setHelp('This command does something'); } protected function execute(InputInterface $input, OutputInterface $output) { $output->writeln('Executing my command...'); // Your command logic goes here return Command::SUCCESS; } } $command = new MyCommand(); $application = new \Symfony\Component\Console\Application(); $application->add($command); $application->run(); ?>
Run the command:
php myCommand.php
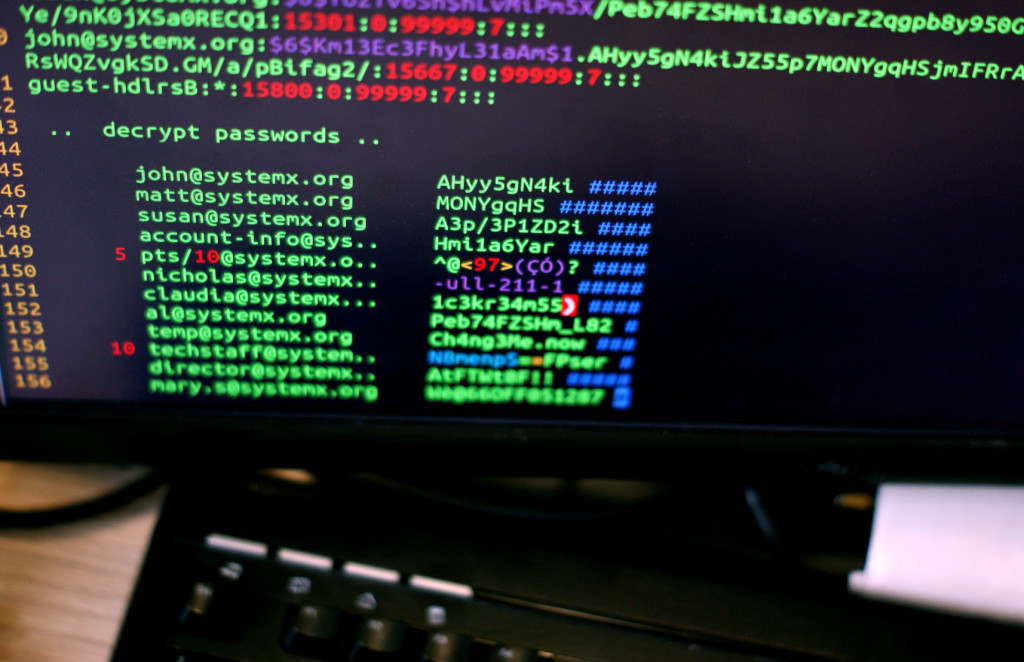
Conclusion
The PHP Command Line Interface offers advanced features for developing powerful scripts and command line tools. From error handling and asynchronous programming to interacting with APIs and parallel processing, these techniques empower developers to create efficient and versatile solutions. Experiment with these advanced concepts to enhance your PHP CLI scripting capabilities.