SOAP (Simple Object Access Protocol) is a protocol for exchanging structured information in web services. PHP provides built-in functionalities for creating and consuming SOAP services. In this article, we’ll explore the process of building and consuming SOAP services in PHP with step-by-step examples.
Part 1: Creating a Simple SOAP Service
Step 1: Set Up a PHP Server
Create a PHP file to act as the server. Let’s name it soap_server.php
:
<?php // Define a simple SOAP service class CalculatorService { public function add($a, $b) { return $a + $b; } public function subtract($a, $b) { return $a - $b; } } // Create a SOAP server $server = new SoapServer(null, ['uri' => 'http://localhost/soap_server.php']); $server->setClass('CalculatorService'); // Handle SOAP requests $server->handle(); ?>
Step 2: Start the PHP Server
Open a terminal, navigate to the directory containing soap_server.php
, and run the PHP built-in server:
php -S localhost:8000
Your SOAP service is now running at http://localhost:8000/soap_server.php.
Part 2: Consuming the SOAP Service
Step 3: Create a PHP Client
Now, let’s create a PHP client to consume the SOAP service. Create a new file named soap_client.php
:
<?php // Create a SOAP client $client = new SoapClient(null, [ 'location' => 'http://localhost:8000/soap_server.php', 'uri' => 'http://localhost/soap_server.php', ]); // Call the add method on the SOAP service $resultAdd = $client->add(5, 3); echo "Result of adding: $resultAdd\n"; // Call the subtract method on the SOAP service $resultSubtract = $client->subtract(10, 4); echo "Result of subtracting: $resultSubtract\n"; ?>
Step 4: Run the PHP Client
Open a new terminal, navigate to the directory containing soap_client.php
, and run the PHP script:
php soap_client.php
You should see the results of the SOAP service operations:
Result of adding: 8 Result of subtracting: 6
Congratulations! You have successfully created and consumed a simple SOAP service in PHP.
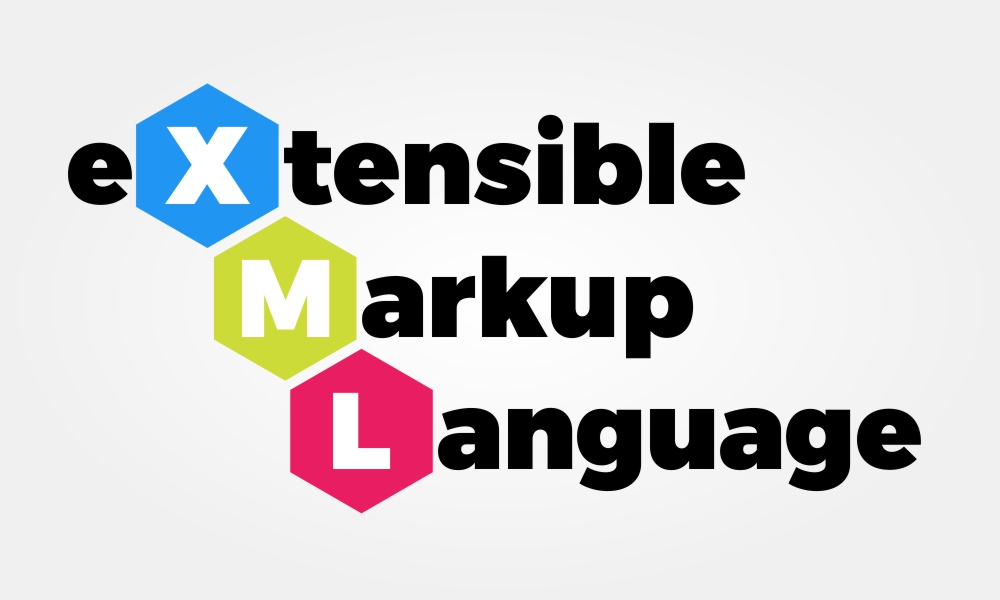
Conclusion
Creating and consuming SOAP services in PHP is a straightforward process. In this guide, we’ve walked through the steps of setting up a PHP SOAP server and consuming it with a PHP client. This is a fundamental example, and in real-world scenarios, you might encounter more complex SOAP services with WSDL (Web Services Description Language) definitions. Understanding the basics provided here will serve as a solid foundation for working with SOAP services in various PHP projects.