Next.js and Nuxt.js are frameworks used for building web applications, but they cater to different ecosystems and have distinct features. Here’s a comparison of the two:
Introduction
Next.js
- Ecosystem: Built for React.js.
- Purpose: Primarily used for building server-rendered React applications.
- Routing: Uses file-based routing with the
pages
directory. Each file in thepages
directory corresponds to a route. - Data Fetching: Offers various data fetching methods such as
getStaticProps
,getServerSideProps
, andgetInitialProps
. - Static Site Generation: Supports both Static Site Generation (SSG) and Server-Side Rendering (SSR).
- Customization: Provides more flexibility and requires more configuration, suitable for advanced use cases.
- Plugins: Does not have a centralized plugin system but allows for using a wide range of npm packages and middleware.
- Community and Support: Large community with extensive documentation and a wide range of resources available.
Nuxt.js
- Ecosystem: Built for Vue.js.
- Purpose: Used for building server-rendered Vue applications and also supports static site generation.
- Routing: Uses file-based routing with the
pages
directory. Similar to Next.js, each file in thepages
directory corresponds to a route. - Data Fetching: Provides an
asyncData
method to fetch data before rendering the page. - Static Site Generation: Supports Static Site Generation (SSG) and Server-Side Rendering (SSR).
- Customization: Provides a more opinionated setup with sensible defaults, making it easier to get started with but potentially less flexible for advanced customization.
- Plugins: Has a built-in plugin system that makes it easy to extend the functionality of your application.
- Community and Support: Strong community with good documentation and support for Vue.js developers.
Next.js is a React-based framework focused on flexibility and extensive customization, ideal for developers who prefer React and need to build highly customized applications.
Nuxt.js is a Vue-based framework with a more opinionated approach, making it easier to get started with Vue projects while still providing powerful features for building both static and server-rendered applications.
When comparing the speed of Next.js and Nuxt.js, it’s important to consider various aspects such as build speed, runtime performance, and static site generation. Both frameworks are designed to be performant, but there are some differences based on their underlying technologies and optimizations:
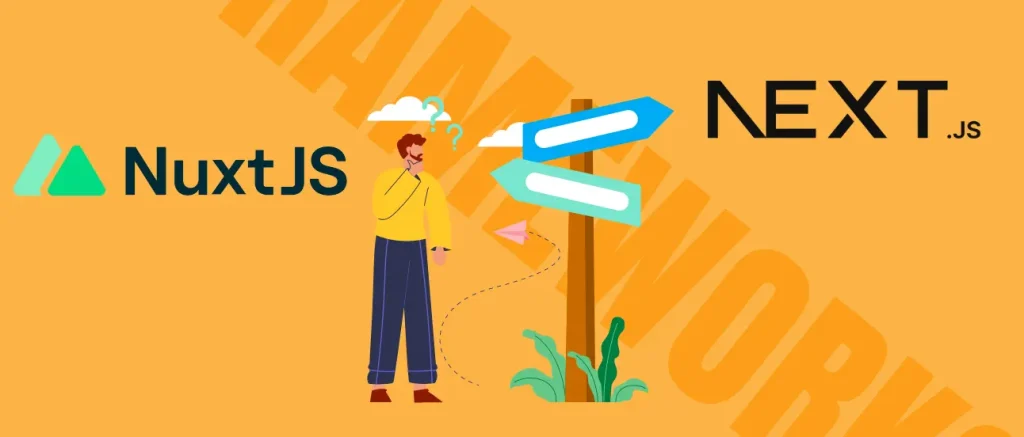
Build Speed
- Next.js: Next.js typically has faster build times for large projects due to its efficient build process and the extensive optimization done by Vercel, the company behind Next.js. It leverages modern build tools like Webpack (or optionally, Vite) to optimize the build process.
- Nuxt.js: Nuxt.js also offers good build performance, especially with recent updates. It uses Webpack for its build process and has incorporated various performance optimizations over time. However, for very large projects, Next.js might have a slight edge in build speed.
Runtime Performance
- Next.js: Next.js is known for its excellent runtime performance, thanks to features like automatic static optimization, code-splitting, and efficient server-side rendering. It also benefits from React’s virtual DOM, which can offer fast updates and rendering.
- Nuxt.js: Nuxt.js also provides strong runtime performance, with efficient server-side rendering and static site generation capabilities. Vue’s reactive system ensures that updates are fast and efficient. Recent improvements in Nuxt 3 and Vue 3 have further enhanced performance, making it comparable to Next.js.
Static Site Generation
- Next.js: Next.js excels in static site generation (SSG) with features like
getStaticProps
and incremental static regeneration (ISR), which allows for high-performance static sites with the ability to update content dynamically. - Nuxt.js: Nuxt.js offers static site generation through
nuxt generate
and also supports incremental static regeneration with its newer versions. The performance is generally very good, but the maturity and widespread use of Next.js for SSG might give it a slight edge.
Development Speed
- Next.js: The development server in Next.js is very fast, with features like fast refresh providing an efficient development experience.
- Nuxt.js: Nuxt.js also offers a quick development server with hot module replacement (HMR) for a smooth development experience.
Performance Optimization Features
- Next.js: Offers features like automatic static optimization, image optimization (next/image), and built-in performance monitoring tools through Vercel.
- Nuxt.js: Provides various performance optimization features such as automatic component-level caching, image optimization, and built-in performance profiling tools.
Both Next.js and Nuxt.js are highly performant frameworks with optimizations for build speed, runtime performance, and static site generation. While Next.js might have a slight advantage in build speed and is well-regarded for its static site generation capabilities, Nuxt.js has made significant strides in performance, especially with Nuxt 3 and Vue 3. The differences in speed are generally marginal and often come down to specific project requirements and preferences for React or Vue ecosystems.
The folder structures of Next.js and Nuxt.js are designed to help developers organize their projects efficiently. Here are the typical folder structures for each:
Next.js Folder Structure
In a standard Next.js project, the folder structure usually looks like this:
my-next-app/ ├── components/ │ ├── Header.js │ └── Footer.js ├── pages/ │ ├── index.js │ ├── about.js │ └── _app.js ├── public/ │ └── images/ │ └── logo.png ├── styles/ │ ├── globals.css │ └── Home.module.css ├── .env.local ├── .gitignore ├── next.config.js ├── package.json └── README.md
Key Directories and Files:
- components/: Contains reusable React components.
- pages/: Contains the application’s pages. Each file corresponds to a route based on its filename.
- public/: Stores static assets such as images, which can be referenced directly in the application.
- styles/: Contains global and modular CSS files.
- .env.local: Stores environment variables.
- next.config.js: Configuration file for customizing the Next.js setup.
- package.json: Manages project dependencies and scripts.
Nuxt.js Folder Structure
A typical Nuxt.js project has a different but similarly organized structure:
my-nuxt-app/ ├── assets/ │ └── logo.png ├── components/ │ ├── Header.vue │ └── Footer.vue ├── layouts/ │ ├── default.vue │ └── error.vue ├── middleware/ │ └── auth.js ├── pages/ │ ├── index.vue │ ├── about.vue │ └── _slug.vue ├── plugins/ │ └── axios.js ├── static/ │ └── favicon.ico ├── store/ │ └── index.js ├── nuxt.config.js ├── package.json └── README.md
Key Directories and Files:
- assets/: Contains uncompiled assets such as Sass, LESS, or images.
- components/: Contains reusable Vue components.
- layouts/: Defines application layouts. Each layout is a Vue component used to wrap pages.
- middleware/: Stores custom middleware functions to run before rendering a page or a group of pages.
- pages/: Contains the application’s pages. Each file corresponds to a route based on its filename.
- plugins/: Stores JavaScript plugins to run before instantiating the root Vue.js application.
- static/: Stores static files that are directly served at the root of the application.
- store/: Contains Vuex store files for state management.
- nuxt.config.js: Configuration file for customizing the Nuxt.js setup.
- package.json: Manages project dependencies and scripts.
Next.js emphasizes a simpler structure with a focus on React components, pages, and static assets.
Nuxt.js provides a more structured setup that includes directories for layouts, middleware, plugins, and store, reflecting its opinionated nature and focus on comprehensive project organization.
Both structures aim to keep the project organized and maintainable, but Nuxt.js offers more built-in conventions, which can help streamline the development process, especially for larger applications.
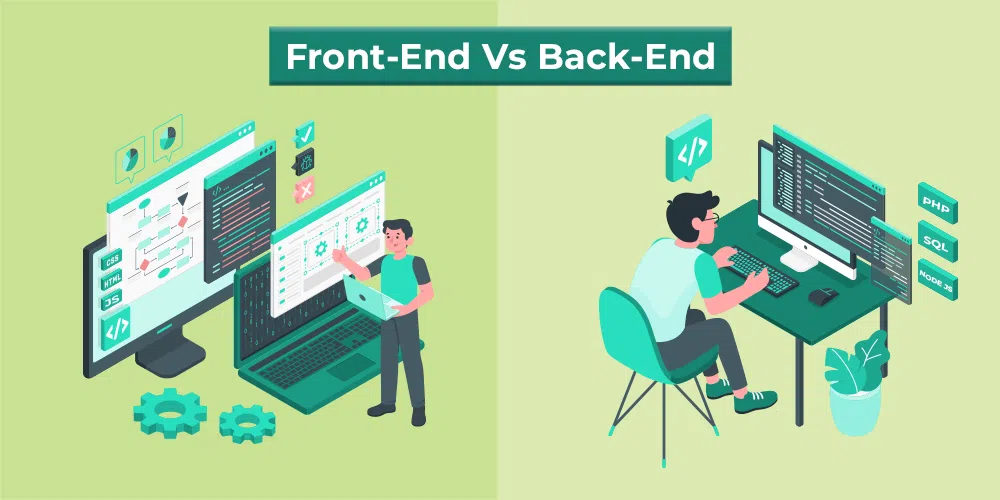
Next.js and Nuxt.js are not back-end frameworks
but they have features that allow for some back-end-like functionality. They are primarily front-end frameworks used for building web applications with React and Vue.js, respectively. However, both frameworks support server-side rendering (SSR) and can handle some server-side logic.
Next.js
- Frontend Framework: Next.js is primarily a framework for building front-end applications with React.
- Server-Side Rendering: Next.js supports SSR, allowing you to render pages on the server before sending them to the client. This can improve performance and SEO.
- API Routes: Next.js provides a way to create API endpoints using file-based routing under the
pages/api
directory. These API routes can be used to handle server-side logic, such as fetching data from a database or processing form submissions. - Deployment: Next.js can be deployed on various platforms, including Vercel, which supports serverless functions, allowing you to run server-side code without managing a server.
Nuxt.js
- Frontend Framework: Nuxt.js is primarily a framework for building front-end applications with Vue.js.
- Server-Side Rendering: Nuxt.js supports SSR, allowing you to render pages on the server, similar to Next.js. This enhances performance and SEO.
- Server Middleware: Nuxt.js allows you to create server middleware to handle server-side logic. This is done using the
serverMiddleware
property in thenuxt.config.js
file. - API Routes: While Nuxt.js doesn’t provide a built-in way to create API routes like Next.js, you can use server middleware to handle API requests.
- Deployment: Nuxt.js can be deployed on various platforms, including those that support serverless functions or traditional servers.
Next.js and Nuxt.js are both front-end frameworks that support server-side rendering and can handle some server-side logic.
Next.js offers built-in API routes, making it easier to create back-end endpoints within the same project.
Nuxt.js allows for server middleware to handle server-side logic but doesn’t have a built-in API route system like Next.js.
While they can handle some back-end tasks, for more complex server-side logic, database interactions, and full-fledged APIs, you would typically use a dedicated back-end framework or service (such as Express.js, Django, or a cloud-based service like Firebase) in conjunction with Next.js or Nuxt.js.
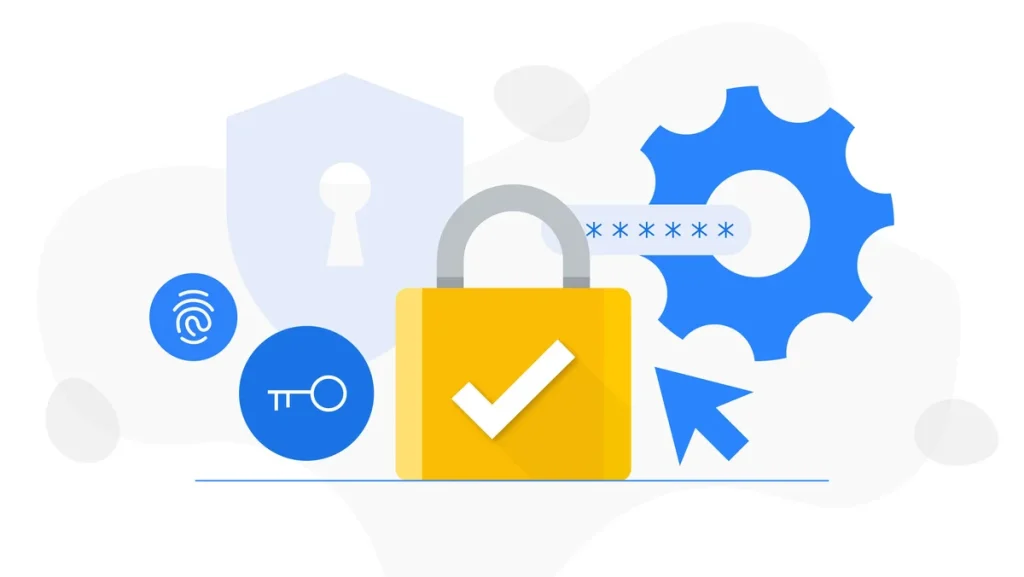
Both Next.js and Nuxt.js have Robust Support for Implementing Security Measures and Authentication.
However, the approach and tools available can differ due to their underlying ecosystems (React for Next.js and Vue.js for Nuxt.js). Here’s an overview of how each framework handles security and authentication:
Next.js
Security:
- Built-in Features: Next.js has several built-in security features such as automatic XSS protection by default in the React ecosystem, Content Security Policy (CSP) support, and prevention of directory traversal attacks.
- Headers Configuration: You can customize HTTP headers for additional security using
next.config.js
to set up headers likeStrict-Transport-Security
,X-Frame-Options
, and others. - Environment Variables: Next.js supports environment variables to securely manage secrets and API keys without exposing them in the client-side code.
Authentication:
- NextAuth.js: A popular library for handling authentication in Next.js. It supports various authentication methods, including OAuth providers (like Google, GitHub), email/password, and custom credentials.
- JWT (JSON Web Tokens): Often used for stateless authentication. Libraries like
jsonwebtoken
can be used in conjunction with API routes. - Session Management: Can be handled using cookies or sessions. The
iron-session
library is commonly used for managing sessions securely. - Middleware: Custom middleware can be added to handle authentication and authorization logic, ensuring only authenticated users can access certain routes.
Nuxt.js
Security:
- Built-in Features: Nuxt.js has built-in support for several security best practices, including automatic XSS protection by default in the Vue.js ecosystem.
- Headers Configuration: Similar to Next.js, you can configure security headers using the
nuxt.config.js
file. - Environment Variables: Nuxt.js supports environment variables for securely managing sensitive information.
Authentication:
- @nuxt/auth-next: A comprehensive module for authentication in Nuxt.js. It supports various authentication strategies, including local authentication (username/password), OAuth2, and social providers (like Google, Facebook).
- JWT (JSON Web Tokens): Can be used for stateless authentication. The
jsonwebtoken
library is also commonly used in Nuxt.js projects. - Session Management: Handled via cookies or sessions, with libraries like
cookie-universal-nuxt
for managing cookies securely. - Middleware: Nuxt.js allows the creation of custom middleware to handle authentication and authorization, ensuring protected routes are only accessible by authenticated users.
Briefly
- Next.js and Nuxt.js both offer strong support for implementing security measures and authentication in web applications.
- Next.js uses libraries like NextAuth.js and
iron-session
for authentication and session management. - Nuxt.js uses the
@nuxt/auth-next
module andcookie-universal-nuxt
for similar purposes. - Both frameworks allow for customization of HTTP headers and secure handling of environment variables to enhance security.
- Middleware in both frameworks can be used to implement custom authentication and authorization logic.
Choosing between the two for security and authentication largely depends on your preference for the React or Vue.js ecosystem and the specific requirements of your project.
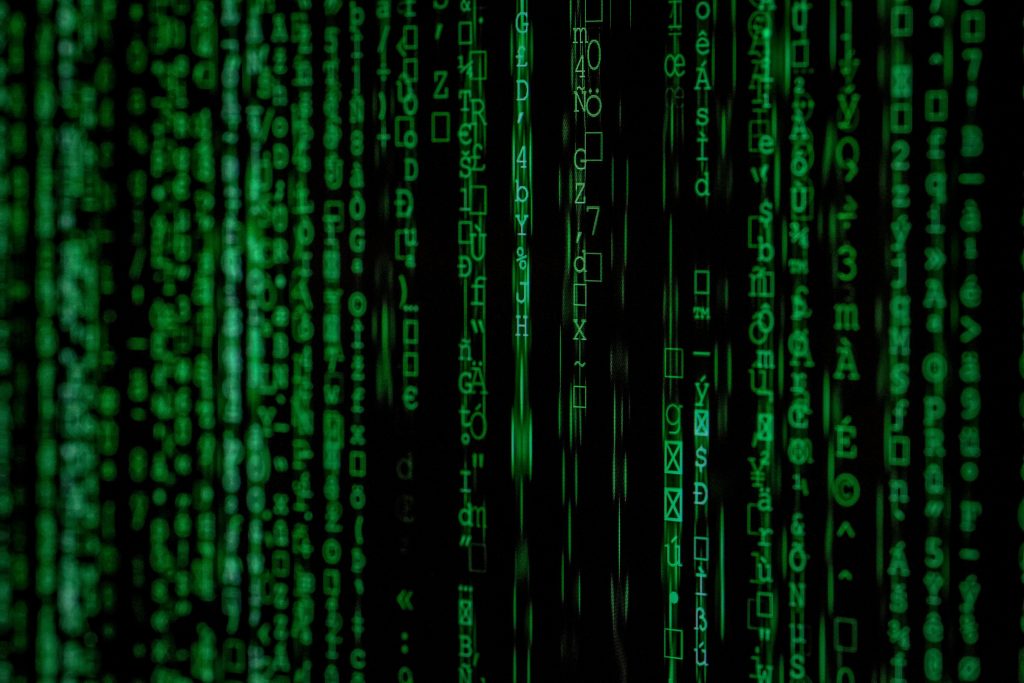
Sample Code of Next.js and Nuxt.js
Sure, here are simple examples of how to set up authentication in both Next.js and Nuxt.js. Each example includes basic project setup and demonstrates a simple authentication flow.
Next.js Sample Source Code
- Project Setup
npx create-next-app my-next-app cd my-next-app npm install next-auth
- pages/api/auth/[…nextauth].js (NextAuth.js configuration)
import NextAuth from 'next-auth'; import Providers from 'next-auth/providers'; export default NextAuth({ providers: [ Providers.GitHub({ clientId: process.env.GITHUB_CLIENT_ID, clientSecret: process.env.GITHUB_CLIENT_SECRET, }), ], database: process.env.DATABASE_URL, });
- pages/_app.js (Wrap your app with the SessionProvider)
import { Provider } from 'next-auth/client'; import '../styles/globals.css'; function MyApp({ Component, pageProps }) { return ( <Provider session={pageProps.session}> <Component {...pageProps} /> </Provider> ); } export default MyApp;
- pages/index.js (Main page with sign-in and sign-out)
import { useSession, signIn, signOut } from 'next-auth/client'; export default function Home() { const [session, loading] = useSession(); return ( <div> {!session && ( <> Not signed in <br /> <button onClick={() => signIn()}>Sign in</button> </> )} {session && ( <> Signed in as {session.user.email} <br /> <button onClick={() => signOut()}>Sign out</button> </> )} </div> ); }
- .env.local (Environment variables)
GITHUB_CLIENT_ID=your-github-client-id GITHUB_CLIENT_SECRET=your-github-client-secret DATABASE_URL=your-database-url
Nuxt.js Sample Source Code
- Project Setup
npx create-nuxt-app my-nuxt-app cd my-nuxt-app npm install @nuxtjs/auth-next @nuxtjs/axios
- nuxt.config.js (Nuxt.js configuration)
export default { modules: ['@nuxtjs/axios', '@nuxtjs/auth-next'], axios: { baseURL: 'http://localhost:3000', }, auth: { strategies: { local: { token: { property: 'token', global: true, required: true, type: 'Bearer' }, user: { property: 'user', autoFetch: true }, endpoints: { login: { url: '/api/auth/login', method: 'post' }, logout: { url: '/api/auth/logout', method: 'post' }, user: { url: '/api/auth/user', method: 'get' } } } } } }
- middleware/auth.js (Middleware for authentication)
export default function ({ store, redirect }) { if (!store.state.auth.loggedIn) { return redirect('/login'); } }
- pages/login.vue (Login page)
<template> <div> <h1>Login</h1> <form @submit.prevent="login"> <input type="text" v-model="username" placeholder="Username" /> <input type="password" v-model="password" placeholder="Password" /> <button type="submit">Login</button> </form> </div> </template> <script> export default { data() { return { username: '', password: '' }; }, methods: { async login() { try { await this.$auth.loginWith('local', { data: { username: this.username, password: this.password } }); this.$router.push('/'); } catch (e) { console.error(e); } } } }; </script>
- pages/index.vue (Protected page)
<template> <div> <h1>Welcome, {{ user.username }}</h1> <button @click="$auth.logout()">Logout</button> </div> </template> <script> export default { middleware: 'auth', computed: { user() { return this.$auth.user; } } }; </script>
- server/api/auth.js (Mock API for authentication)
const express = require('express'); const app = express(); app.use(express.json()); const users = [ { id: 1, username: 'user1', password: 'pass1' }, { id: 2, username: 'user2', password: 'pass2' } ]; app.post('/api/auth/login', (req, res) => { const user = users.find( u => u.username === req.body.username && u.password === req.body.password ); if (user) { res.json({ token: 'fake-jwt-token', user }); } else { res.status(401).json({ message: 'Invalid credentials' }); } }); app.post('/api/auth/logout', (req, res) => { res.json({ message: 'Logged out' }); }); app.get('/api/auth/user', (req, res) => { res.json({ user: users[0] }); }); module.exports = app;
- nuxt.config.js (Server middleware)
export default { serverMiddleware: [ { path: '/api/auth', handler: '~/server/api/auth.js' } ], ... }
These examples demonstrate basic authentication flows in both Next.js and Nuxt.js. In real-world applications, you would likely connect to a real back-end service for user authentication and handle more sophisticated security measures.